GoLang API 开发简介
GoLangAPIGo
https://blog.octo.com/en/hexagonal-architecture-three-principles-and-an-implementation-example/
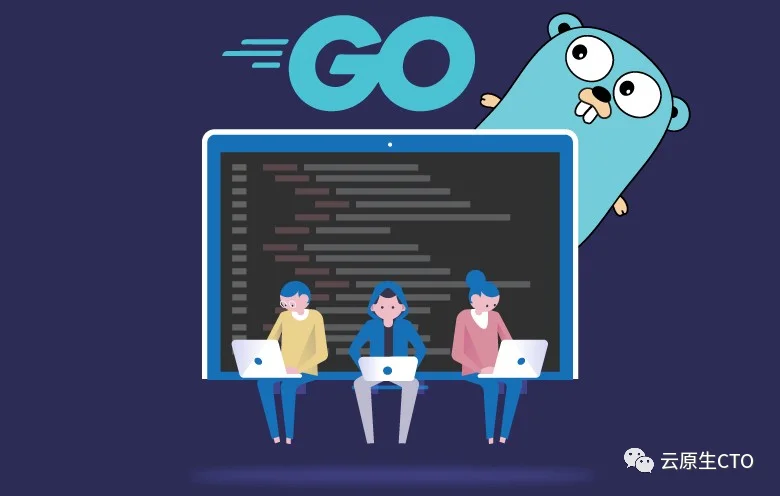
GoLangGithub
让我们现在开始
APIDB
Fresh
go get github.com/pilu/fresh
mod
go mod init github.com/jainam240101/todo
添加我们将在整个应用程序中使用的这些库
go get github.com/dgrijalva/jwt-go github.com/gin-gonic/gin github.com/google/uuid gorm.io/gorm gorm.io/driver/postgres golang.org/x/crypto/bcrypt
文件夹结构
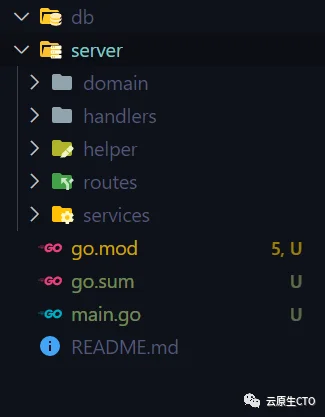
UserModelUsersUserRepositoryDb.goUsers.go
package users
import (
"github.com/google/uuid"
"gorm.io/gorm"
)
type UserModel struct {
gorm.Model
ID uuid.UUID `gorm:"type:char(36);primary_key"`
Name string `json:"name"`
Email string `json:"email" gorm:"uniqueIndex"`
Password string `json:"password"`
Username string `json:"username" gorm:"uniqueIndex"`
}
type UserRepository interface {
CreateUser(UserModel) (*UserModel, error)
}
view raw
Users.gogormGORMcreated_atupdated_atdeleted_atUniqueIndex
package users
import (
"gorm.io/gorm"
)
type UserRepositoryDb struct {
Client *gorm.DB
}
func NewUserRepositoryDb(client *gorm.DB) UserRepositoryDb {
return UserRepositoryDb{Client: client}
}
func (d UserRepositoryDb) CreateUser(u UserModel) (*UserModel, error) {
if err := d.Client.Save(&u).Error; err != nil {
return nil,err
}
return &u, nil
}
view raw
UserService.go
package users
import (
"github.com/google/uuid"
users "github.com/jainam240101/todo/server/domain/Users"
"golang.org/x/crypto/bcrypt"
)
type DefaultUserService struct {
repo users.UserRepositoryDb
}
type UserService interface {
CreateUser(users.UserModel) (*users.UserModel, error)
}
func NewCustomerService(repository users.UserRepositoryDb) DefaultUserService {
return DefaultUserService{repo: repository}
}
func HashPassword(password string) (string, error) {
bytes, err := bcrypt.GenerateFromPassword([]byte(password), 4)
if err != nil {
return "", err
}
return string(bytes), nil
}
func (db DefaultUserService) CreateUser(u users.UserModel) (*users.UserModel, error) {
pass, err := HashPassword(u.Password)
if err != nil {
return nil, err
}
u.Password = pass
u.ID = uuid.New()
user, err := db.repo.CreateUser(u)
if err != nil {
return nil, err
}
return user, nil
}
NewCustomerServiceUserRepository
API
package users
import (
"github.com/gin-gonic/gin"
domain "github.com/jainam240101/todo/server/domain/Users"
service "github.com/jainam240101/todo/server/services"
)
type Userhandlers struct {
Service service.UserService
}
func (uh *Userhandlers) CreateUser(c *gin.Context) {
u := domain.UserModel{}
if err := c.BindJSON(&u); err != nil {
c.JSON(406, gin.H{
"message": "Body Not Proper",
})
return
}
user, err := uh.Service.CreateUser(u)
if err != nil {
c.JSON(406, gin.H{
"message": "Body Not Proper",
})
return
}
c.JSON(200, gin.H{
"data": user,
})
}
view raw
RoutesUserRoutes.go
package routes
import (
"github.com/gin-gonic/gin"
domain "github.com/jainam240101/todo/server/domain/Users"
service "github.com/jainam240101/todo/server/services"
handlers "github.com/jainam240101/todo/server/handlers"
"gorm.io/gorm"
)
func UserRoutes(apiRouter *gin.RouterGroup, db *gorm.DB) {
uh := handlers.Userhandlers{Service: service.NewCustomerService(domain.NewUserRepositoryDb(db))}
route := apiRouter.Group("/users")
{
route.POST("/", uh.CreateUser)
}
}
12NewUserRepositoryDbNewUserServiceNewUserRepositoryDbNewUserServiceRepositoryDbUser
APImain
package main
import (
"github.com/gin-gonic/gin"
"github.com/jainam240101/todo/db"
Routes "github.com/jainam240101/todo/server/routes"
)
func main() {
db.Init()
router := gin.Default()
Routes.UserRoutes(&router.RouterGroup, db.DB)
router.Run()
}
localhost:8080
fresh
21
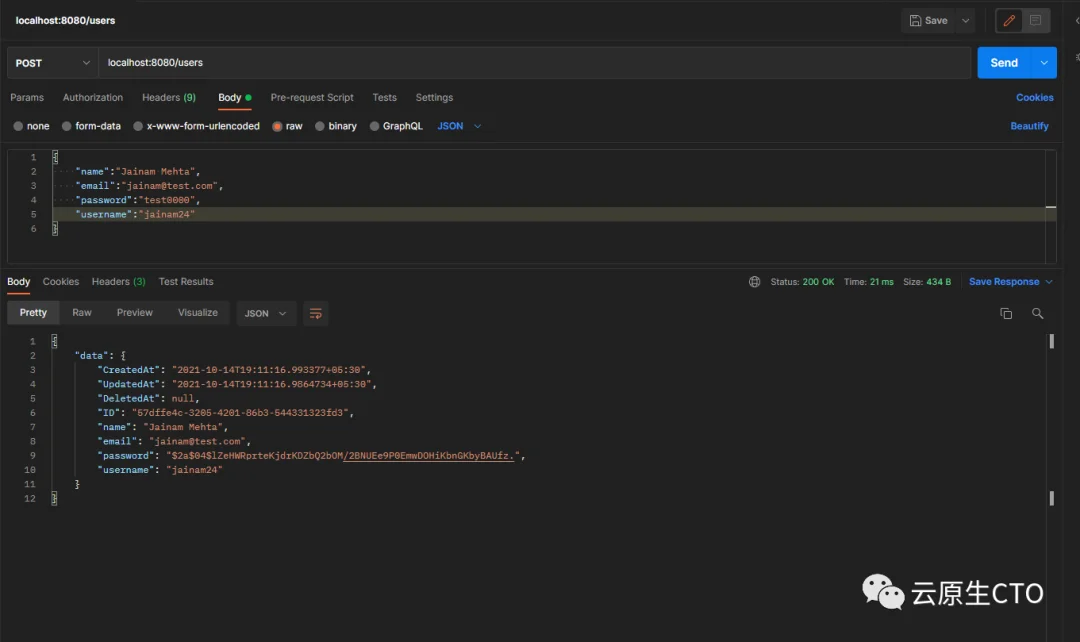
APIGithub
https://github.com/jainam240101/hexagonal-architecture-blog/tree/1-Introduction
进一步阅读[1]
参考资料
参考地址: https://medium.com/@jainamm47/introduction-to-api-development-in-golang-c5dcecc47dd2。