- 基本的介绍
- 时间和日期相关函数,需要导入 time 包
- 参考网址:
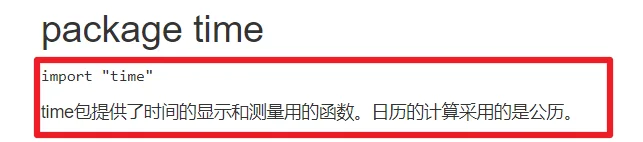
- time.Time 类型,用于表示时间
- 获取当前时间
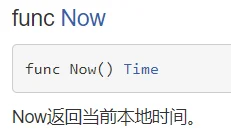
now := time.Now()
fmt.Printf("now=%v now type=%T\n", now, now)

- 获取到日期信息,通过now可以获取到年月日,时分秒
fmt.Printf("年=%v\n", now.Year())
fmt.Printf("月=%v\n", now.Month())
fmt.Printf("月=%v\n", int(now.Month()))
fmt.Printf("日=%v\n", now.Day())
fmt.Printf("时=%v\n", now.Hour())
fmt.Printf("分=%v\n", now.Minute())
fmt.Printf("秒=%v\n", now.Second())
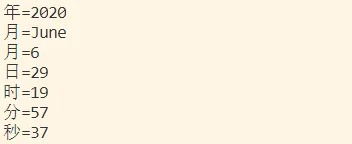
- 格式化日期时间
- 方式 1: 就是使用 Printf 或者 SPrintf
fmt.Printf("当前年月日 %d-%d-%d %d:%d:%d \n", now.Year(),
now.Month(), now.Day(), now.Hour(), now.Minute(), now.Second())
dateStr := fmt.Sprintf("当前年月日 %d-%d-%d %d:%d:%d \n", now.Year(),
now.Month(), now.Day(), now.Hour(), now.Minute(), now.Second())
fmt.Printf("dateStr=%v\n", dateStr)

- 方式二: 使用 time.Format() 方法完成:
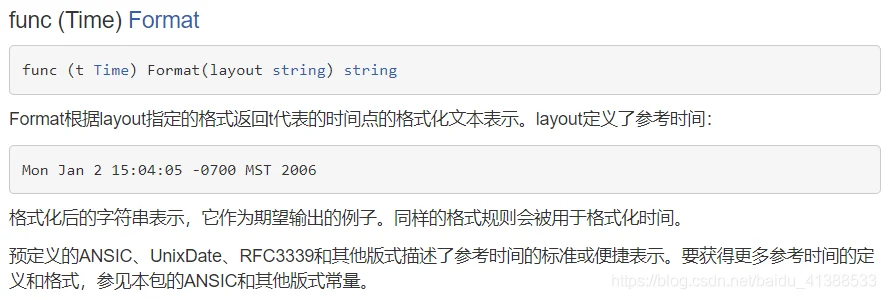
fmt.Printf(now.Format("2006-01-02 15:04:05"))
fmt.Println()
fmt.Printf(now.Format("2006-01-02"))
fmt.Println()
fmt.Printf(now.Format("15:04:05"))
fmt.Println()
fmt.Printf(now.Format("2006"))
fmt.Println()

- "2006/01/02 15:04:05" 这个字符串的各个数字是固定的,必须是这样写。
- "2006/01/02 15:04:05" 这个字符串各个数字可以自由的组合,这样可以按程序需求来返回时间和日期
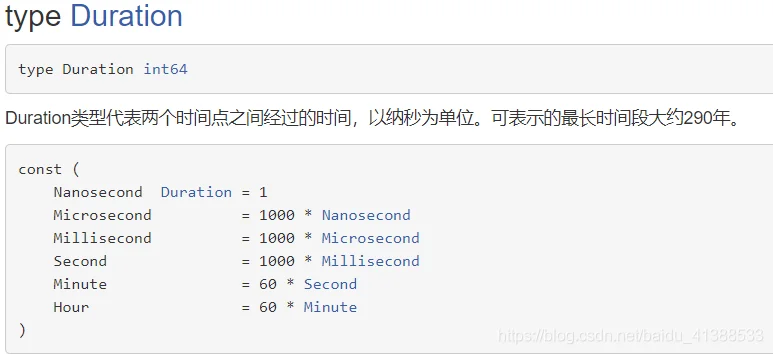
const (
Nanosecond Duration = 1 -- 纳秒
Microsecond = 1000 * Nanosecond -- 微秒
Millisecond = 1000 * Microsecond -- 毫秒
Second = 1000 * Millisecond -- 秒
Minute = 60 * Second -- 分钟
Hour = 60 * Minute --小时
)
- 常量的作用:在程序中可用于获取指定时间单位的时间,比如想得到 100 毫秒
i := 0
for {
i++
fmt.Println(i)
//休眠
time.Sleep(time.Second)
if i == 100 {
break
}
}
- 每隔0.1秒中打印一个数字,打印到100时就退出,不能用 time.Second * 0.1
i := 0
for {
i++
fmt.Println(i)
time.Sleep(time.Millisecond * 100)
if i == 100 {
break
}
}
- time 的 Unix 和 UnixNano 的方法 可以用来获取随机数字
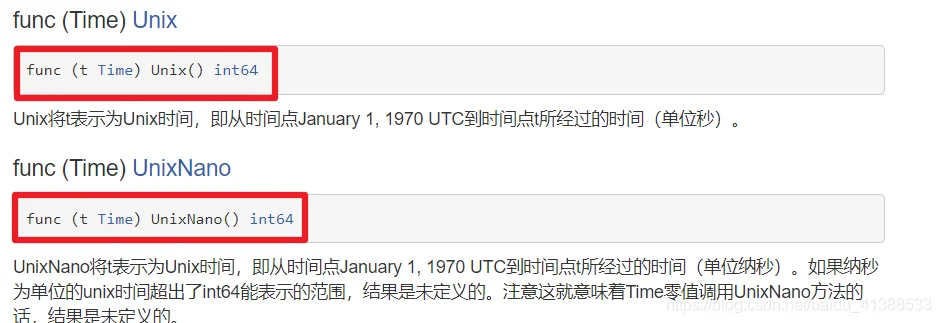
fmt.Printf("unix时间戳=%v unixnano时间戳=%v\n", now.Unix(), now.UnixNano())

- 演示案例:
- 编写一段代码来统计 函数 test03 执行的时间
package main
import (
"fmt"
"time"
"strconv"
)
func test03() {
str := ""
for i := 0; i < 100000; i++ {
str += "hello" + strconv.Itoa(i)
}
}
func main() {
//在执行test03前,先获取到当前的unix时间戳
start := time.Now().Unix()
test03()
end := time.Now().Unix()
fmt.Printf("执行test03()耗费时间为%v秒\n", end-start)
}
